(18)FUNCTION ARGUMENTS:
1.Difference between parameters and arguments:
Parameter are variables used in parentheses while defining a function.
Arguments are values passed for the parameter while calling a function.
In the following example name is the parameter and 'Alex' is the argument.
for example:
def print_name(name):
print(name)
print_name('Alex')
output = Alex
2.positional and keyword arguments
The arguments can be positional arguments or keyword arguments.
Positional arguments are the arguments that are given by the position of the parameter.
Keyword arguments are the arguments that are given by setting the variable equal to the parameter.
for example:
def foo(a, b, c):
print(a, b, c)
#positional argument
foo(1, 2, 3)
#keyword argument
foo(c=3, b=2, a=1)
foo(1, b=2, c=3)
output =
1 2 3
1 2 3
1 2 3
3.Default arguments:
Default arguments are the arguments which can store two different values in the same variable.
important : Default arguments must be at the end.
for example:
def foo(a, b, c, d=4):
print(a, b, c , d)
foo(1, 2, 3, 4)
foo(1, b=2, c=3, d=100)
output = 1 2 3 4
1 2 3 100
4.Variable-length arguments(*args and **kwargs)
*args: marking asterisk(*) to a parameter means that you can pass any number positional argument to it. It is not necessary to always write args after asterisk(*) you can write any variable you want but the main thing is that you type asterisk(*) before naming that is what defines *args the positional argument. The same goes for **kwargs.
**kwargs: marking asterisk(**) to a parameter means that you can pass any number of keyword argument to it.
for example:
def foo(a, b, *args, **kwargs):
print(a, b)
# arg means every element in the positional argument in args
for arg in args:
print(arg)
# k means every element in the keyword argument in kwargs
for k in kwargs:
print(k, kwargs[k])
# 3, 4, 5 are combined into args
# six and seven are combined into kwargs
foo(1, 2, 3, 4, 5, six=6, seven=7)
print()
# omitting of args or kwargs is also possible
foo(1, 2, three=3)
output =
1 2
3
4
5
six 6
seven 7
1 2
three 3
5.Forced keyword arguments
You can also force only to give keyword argument to parameter without using **kwargs by putting (*) before the function parameter list
for example:
def foo(a, b, *, c, d):
print(a, b, c, d)
foo(1, 2, c=3, d=4)
def foo(*args, last):
for arg in args:
print(arg)
print(last)
foo(8, 9, 10 , last=50)
output =
1 2 3 4
8
9
10
50
6.Unpacking into arguments
List or tuples can be unpacked by typing( *) before calling the function with the variable in which the tuple or the list is stored.
To unpack dictionaries put (**) before calling the function with the variable in which the dictionary is stored.
for example:
def foo(a, b, c):
print(a, b, c)
mylist = [4, 5, 6]
foo(*mylist)
mydict = {'a': 1, 'b': 2, 'c': 3}
foo(**mydict)
output =
4 5 6
1 2 3
7.Local and global variables
local variables can only be used in the function not outside the function. To disable this you can use global before typing the name of the function.
#global variable_name
for example:
def foo1():
x = number
print('number in function:', x)
number = 0
foo1()
def foo2():
global number
number = 3
print('number before foo2():', number)
foo2()
print('number after foo2():', number)
output =
number in function: 0
number before foo2(): 0
number after foo2(): 3
8.Passing the parameter
In python there is a mechanism, which is called "Call-by-Object" or "Call-by-Object-Reference. The following rules must be considered:
• The parameter passed in is actually a reference to an object.
(Means that mutable objects such as lists or dict can be changed within a method. But if you rebind the outer reference in the method, the outer reference will still point at the original object.)
• Difference between mutable and immutable data types.
(Means that immutable objects such as int or string cannot be changed within a method
but immutable objects contained within a mutable object can be re-assigned within a method)
Immutable
for example:
# immutable objects -> no change
def foo(x):
x = 5 # x += 5 also no effect since x is immutable and a new variable must be created
var = 10
print('var before foo():', var)
foo(var)
print('var after foo():', var)
output =
var before foo(): 10
var after foo(): 10
Mutable
for example:
def foo(a_list):
a_list.append(4)
my_list = [1, 2, 3]
print('my_list before foo():', my_list)
foo(my_list)
print('my_list after foo():', my_list)
output =
my_list before foo(): [1, 2, 3]
my_list after foo(): [1, 2, 3, 4]
Immutable object in a mutable object
for example:
# immutable objects within a mutable object -> change
def foo(a_list):
a_list[0] = -100
a_list[2] = "Paul"
my_list = [1, 2, "Max"]
print('my_list before foo():', my_list)
foo(my_list)
print('my_list after foo():', my_list)
output =
my_list before foo(): [1, 2, 'Max']
my_list after foo(): [-100, 2, 'Paul']
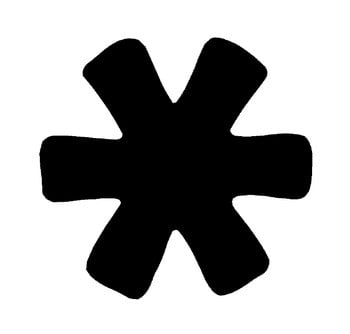
(19)ASTERISK OPERATOR:
The asterisk(*) is used in the multiplication operations, power operations, *args and **kwargs, unpacking list, tuples and dictionary etc.
1.Mutipication.
To do multiplication operation in python.
for example:
result = 5 * 7
print(result)
output = 35
2.Power operations
To raise the number to a power put two asterisk(**) after the number and then type the number which is the power.
for example:
result = 2 ** 4
print(result)
output = 16
3.Printing elements multiple times in list and tuples.
lists and tuples:
for example:
zeros = [0, 1] * 10
print(zeros)
output = [0, 1, 0, 1, 0, 1, 0, 1, 0, 1, 0, 1, 0, 1, 0, 1, 0, 1, 0, 1]
strings:
for example:
string = "AB" * 10
print(string)
output = ABABABABABABABABABAB
4.*args and **kwargs
for example:
def foo(a, b, *args, **kwargs):
print(a, b)
for arg in args:
print(arg)
for key in kwargs:
print(key, kwargs[key])
foo(1, 2, 3, 4, 5, six=6, seven=7)
output =
1 2
3
4
5
six 6
seven 7
5.Unpacking lists, strings and dictionaries
for example:
numbers = (1, 2, 3, 4, 5, 6, 7, 8)
*beginning, last = numbers
print(beginning)
print(last)
print()
first, *end = numbers
print(first)
print(end)
print()
first, *middle, last = numbers
print(first)
print(middle)
print(last)
output =
[1, 2, 3, 4, 5, 6, 7]
8
1
[2, 3, 4, 5, 6, 7, 8]
1
[2, 3, 4, 5, 6, 7]
8
6.Merging list and dictionary
for example:
my_tuples = (1, 2, 3)
my_set = {4, 5, 6}
my_list = [*my_tuples, *my_set]
print(my_list)
dict_a = {'one': 1, 'two': 2}
dict_b = {'three': 3, 'four': 4}
dict_c = {**dict_a, **dict_b}
print(dict_c)
output =
[1, 2, 3, 4, 5, 6]
{'one': 1, 'two': 2, 'three': 3, 'four': 4}
(20)COPYING:
In python there are two types of copying shallow and deep.
shallow copy: Only one level deep. It creates a new collection object and populates it with references to the nested objects. This means modifying a nested object in the copy deeper than one level affects the original.
for example:
import copy
org = [0, 1, 2 , 3, 4]
cpy = copy.copy(org)
cpy[0] = -10
print(cpy)
print(org)
output =
[-10, 1, 2, 3, 4]
[0, 1, 2, 3, 4]
deep copy: A full independent clone.
It creates a new collection object and then recursively populates it with copies of the nested objects found in the
original.
for example:
import copy
org = [[0, 1, 2, 3, 4], [5, 6, 7, 8, 9]]
cpy = copy.deepcopy(org)
cpy[0][1] = -10
print(cpy)
print(org)
output =
[[0, -10, 2, 3, 4], [5, 6, 7, 8, 9]]
[[0, 1, 2, 3, 4], [5, 6, 7, 8, 9]]
1.Custom objects
for example:
import copy
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
# Only copies the reference
class Company:
def __init__(self, boss, employee):
self.boss = boss
self.employee = employee
boss = Person('Jane', 55)
employee = Person('Joe', 28)
company = Company(boss, employee)
company_clone = copy.copy(company)
company_clone.boss.age = 56
print(company.boss.age)
print(company_clone.boss.age)
print()
boss = Person('Jane', 55)
employee = Person('Joe', 28)
company = Company(boss, employee)
company_clone.boss.age = 56
print(company.boss.age)
print(company_clone.boss.age)
output =
56
56
55
56
Comments
Post a Comment
If you have any doubts please let me know